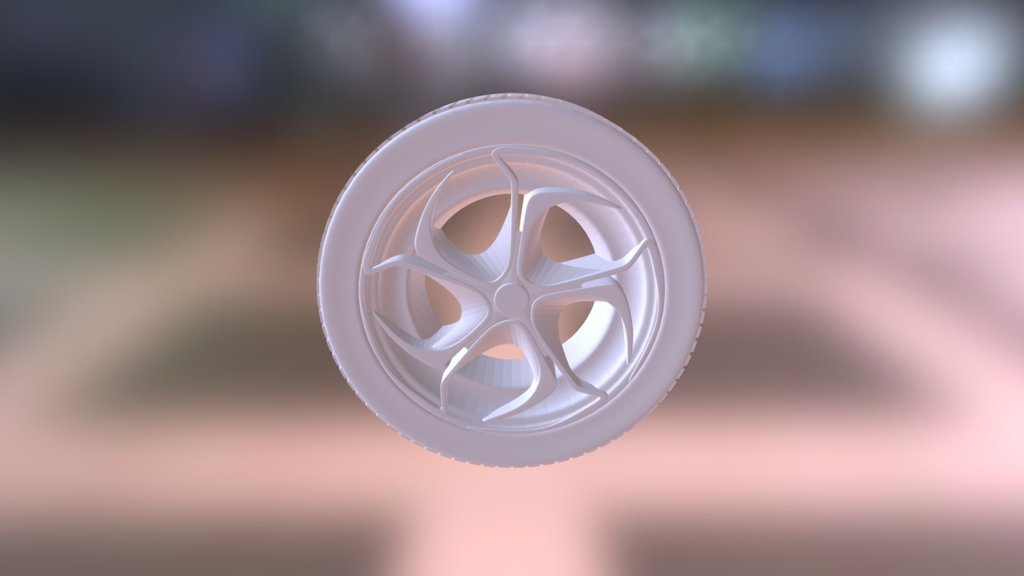
Wheel Model
sketchfab
Modeling A Wheel Exercise We're going to build a real wheel using just code! Imagine you are building a toy car and it needs a wheel. You will learn how to write your own program that generates a 3D wheel from scratch. The goal is not only to create the model but also to understand how 3D modeling works. Let's Start: - Wheel diameter (D): 10 units - Rim width (W): 2 units - Hub radius (H): 1 unit We will use Python and a library called Matplotlib to generate our wheel. First, we need to import the necessary libraries: ```python import matplotlib.pyplot as plt from mpl_toolkits.mplot3d import Axes3D ``` Next, let's define some variables for our wheel dimensions: ```python D = 10 W = 2 H = 1 ``` Now we can draw the wheel. We will use a loop to create each point on the circle and then plot it. ```python fig = plt.figure() ax = fig.add_subplot(111, projection='3d') theta = np.linspace(0, 2*np.pi, 100) x = D/2 * np.cos(theta) + W/2 * np.sin(theta) y = D/2 * np.sin(theta) - W/2 * np.cos(theta) z = H ax.plot(x, y, z) plt.show() ``` The code will create a simple wheel with the specified dimensions. However, our goal is to generate this model from scratch. So let's use Matplotlib's built-in functions to draw the circle. ```python import numpy as np fig = plt.figure() ax = fig.add_subplot(111, projection='3d') theta = np.linspace(0, 2*np.pi, 100) x = D/2 * np.cos(theta) + W/2 * np.sin(theta) y = D/2 * np.sin(theta) - W/2 * np.cos(theta) ax.plot(x, y, z=np.zeros_like(x)) plt.show() ``` Now we have a wheel model that is generated from code. We can adjust the dimensions and parameters to suit our needs. Let's Try Another Approach: We will use Matplotlib's 3D plotting capabilities to draw the wheel. ```python import numpy as np fig = plt.figure() ax = fig.add_subplot(111, projection='3d') theta = np.linspace(0, 2*np.pi, 100) x = D/2 * np.cos(theta) + W/2 * np.sin(theta) y = D/2 * np.sin(theta) - W/2 * np.cos(theta) ax.plot(x, y, z=np.zeros_like(x)) plt.show() ``` This code will generate a wheel model with the specified dimensions.
With this file you will be able to print Wheel Model with your 3D printer. Click on the button and save the file on your computer to work, edit or customize your design. You can also find more 3D designs for printers on Wheel Model.