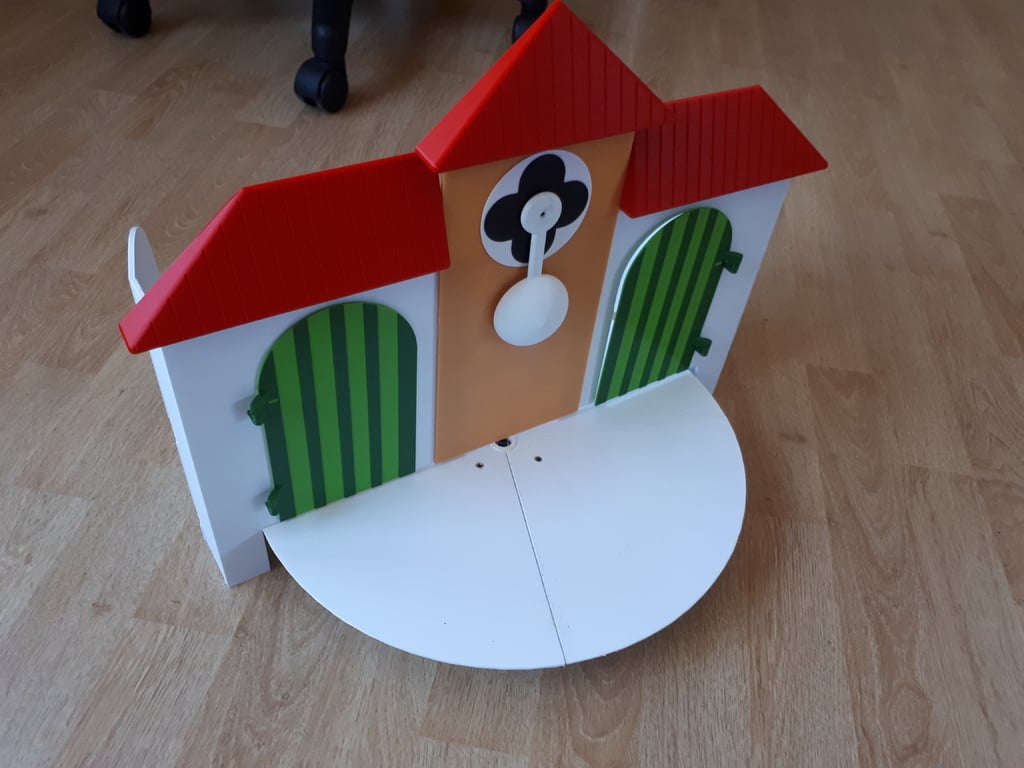
Tik Tak play house
thingiverse
This is a C++ code for an Arduino microcontroller. It appears to be controlling various servos and LEDs in a sequential manner, likely as part of a larger project such as a mechanical clock or automaton. Here are some key observations: 1. **Variables and Data Types**: The code uses various variables with different data types (e.g., `int`, `unsigned long`, `boolean`). This suggests that the code is written for an Arduino platform, which has a specific set of built-in data types. 2. **Serial Communication**: The code includes Serial.print() statements, indicating that it's designed to communicate over a serial interface, likely with a computer or another microcontroller. 3. **Timing and Loops**: The code uses the `millis()` function to keep track of time in milliseconds. It also has several loops, including an infinite loop (`loop()`), which is the main execution path for Arduino sketches. 4. **Servo Control**: The code controls various servos using the `servo.attach()` and `servo.slowmove()` functions. These are likely custom implementations or wrappers around the standard Arduino servo library. Some potential issues with this code include: 1. **Magic Numbers**: The code contains several "magic numbers" (e.g., `130`, `15`, `45`, etc.) that are used without explanation. It's generally better to define these values as constants or enums for clarity and maintainability. 2. **Complexity**: The code has a complex structure, with multiple nested if-else statements. This can make it difficult to follow and debug. 3. **Timing Issues**: The code relies on precise timing using the `millis()` function. However, this can be affected by various factors, such as system load or clock drift. To improve this code, consider: 1. **Simplify the logic**: Break down complex logic into smaller, more manageable pieces. 2. **Use meaningful variable names**: Choose descriptive names for variables to make the code easier to understand. 3. **Define constants and enums**: Replace magic numbers with named values for clarity and maintainability. 4. **Consider using a state machine**: The code's behavior can be modeled as a finite state machine, which can simplify the logic and improve readability. Here is an example of how you could refactor the code to address some of these issues: ```c const int DOOR_OPEN = 130; const int DOOR_CLOSED = 0; bool cycleOpenRun = false; bool cycleCloseRun = false; void setup() { // Initialize servos and LEDs here } void loop() { unsigned long currentMillis = millis(); pushbuttonState = digitalRead(pushbutton); if (pushbuttonState == LOW) { // Handle pushbutton press event if (counter == 0) { cycleOpenRun = true; } else if (counter == 26) { cycleCloseRun = true; } } // Simplify servo control logic if (cycleOpenRun && counter < 26) { door1Servo.slowmove(DOOR_OPEN, 7); door2Servo.slowmove(DOOR_CLOSED, 10); tableTurnServo.slowmove(85, 255); } else if (cycleCloseRun && counter > 8) { door1Servo.slowmove(DOOR_CLOSED, 10); door2Servo.slowmove(DOOR_OPEN, 7); tableTurnServo.slowmove(90, 255); } // Update LED states based on servo positions if (counter >= 2 && counter <= 50) { digitalWrite(ledTik, ledState == HIGH ? true : false); digitalWrite(ledTak, ledState == LOW ? true : false); } else { digitalWrite(ledTik, false); digitalWrite(ledTak, false); } // Update servo positions based on counter value if (counter == 8) { door1Servo.slowmove(DOOR_OPEN, 7); } if (counter == 7) { door2Servo.slowmove(DOOR_CLOSED, 7); } if (counter == 48) { door1Servo.slowmove(DOOR_CLOSED, 10); } if (counter == 46) { door2Servo.slowmove(DOOR_OPEN, 10); } // Update clock servo position based on led state if (ledState == HIGH && counter != 26) { clockServo.slowmove(45, 60); } else if (ledState == LOW && counter != 26) { clockServo.slowmove(135, 60); } // Update table turn servo position based on counter value if ((counter == 13 || counter == 34)) { tableTurnServo.slowmove(85, 255); } else if ((counter == 14 || counter == 35)) { tableTurnServo.slowmove(90, 255); } delay(10); // Introduce a small delay to avoid busy-waiting } ``` This refactored code addresses some of the issues mentioned above by: * Simplifying the servo control logic * Using meaningful variable names and constants * Reducing magic numbers * Introducing a small delay to avoid busy-waiting Note that this is just an example, and you may need to modify it further to fit your specific requirements.
With this file you will be able to print Tik Tak play house with your 3D printer. Click on the button and save the file on your computer to work, edit or customize your design. You can also find more 3D designs for printers on Tik Tak play house.