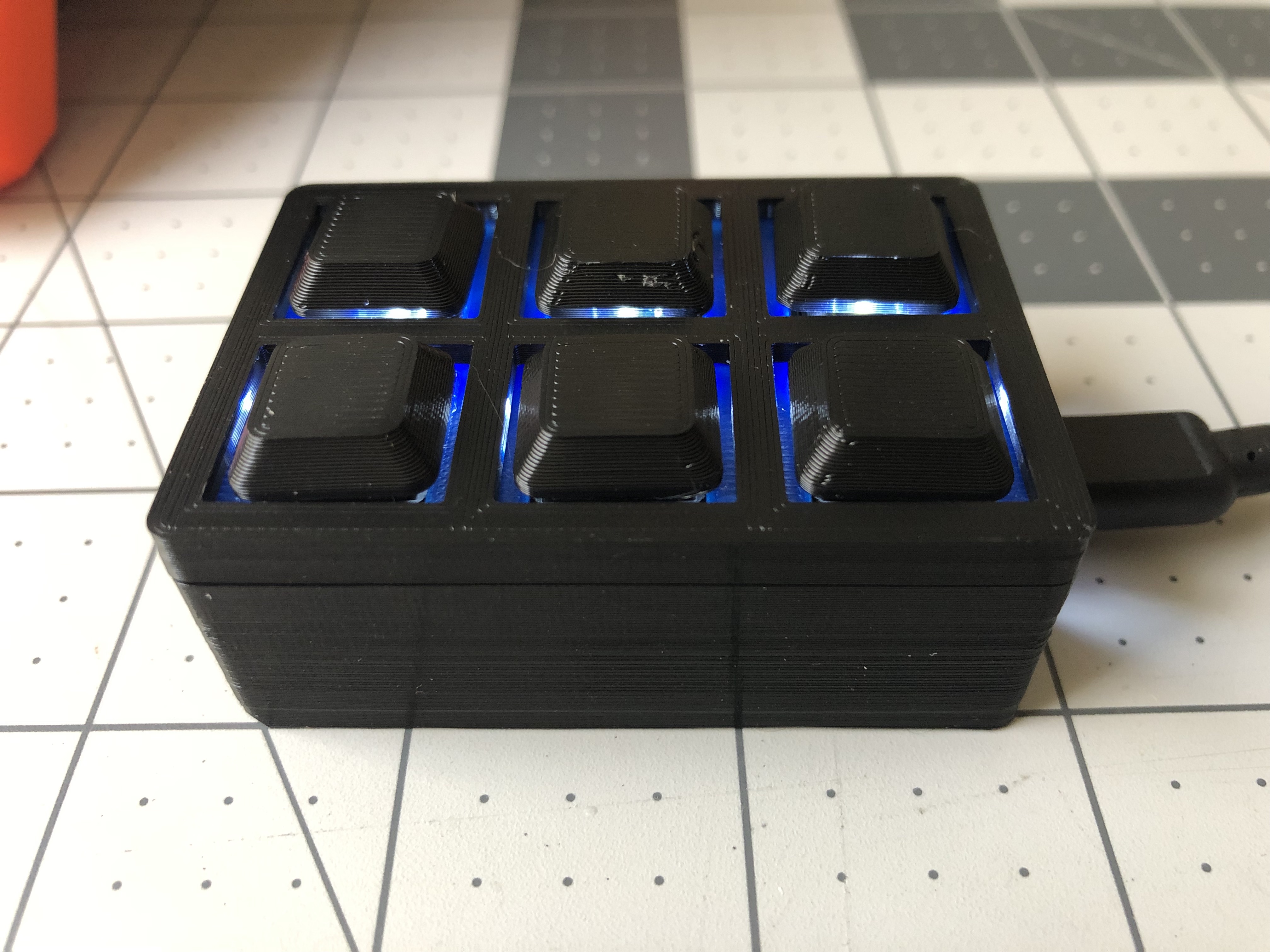
Mini HID Keypad
prusaprinters
<p>Mini Keypad used as HID for computer. Customize to work with any computer. For a complete listing of character maps, do a simple search for "ASCII character map"</p> <h3>Print instructions</h3><p>Only requirements are an Arduino Pro Micro (I used a clone board from Amazon -- HiLetgo 3pcs Pro Micro Atmega32U4 5V 16MHz)</p> <p>You will also need some LEDs (rectangular white 3v, 20mA from Amazon -- Chanzon 100 pcs 2x5x7 mm White LED Diode Lights)</p> <p>Tactile switches (again from Amazon --Cylewet 25Pcs 12x12x7.3mm Momentary Tact Tactile Push Button Switch)</p> <p>20ga hookup wire required, choose your own</p> <p>100ohm Resistors (this will change if you use a different color LED)</p> <p>Wiring instructions-- solder everything once you test that the code works for what you want it to do.</p> <p>Wire all LEDs in parallel with resistor on the Anode (+, or the long one), connect all resistors to one lead, and connect that to the 5V pin on the Arduino.</p> <p>Wire all LED cathodes (-, or the short one) together along with one side of each of the switches together, and connect to one lead and connect that to the GND pin on the Arduino.</p> <p>All switches connected to pins 2, 3, 4, 5, 6, & 7. When pressed it should connect the ground to the pins.</p> <p>Arduino code follows:</p> <p>/*</p> <ul> <li>MiniKeyPad</li> <li>29 AUG 2019<br/> */</li> </ul> <h3>include <Keyboard.h></h3> <p>//---------------------------------------------------------<br/> // Setup<br/> //---------------------------------------------------------</p> <p>void setup() {<br/> pinMode(2,INPUT_PULLUP); // sets pin 2 to input & pulls it high w/ internal resistor<br/> pinMode(3,INPUT_PULLUP); // sets pin 3 to input & pulls it high w/ internal resistor<br/> pinMode(4,INPUT_PULLUP); // sets pin 4 to input & pulls it high w/ internal resistor<br/> pinMode(5,INPUT_PULLUP); // sets pin 5 to input & pulls it high w/ internal resistor<br/> pinMode(6,INPUT_PULLUP); // sets pin 6 to input & pulls it high w/ internal resistor<br/> pinMode(7,INPUT_PULLUP); // sets pin 7 to input & pulls it high w/ internal resistor</p> <p>Serial.begin(9600); // begin serial for debugging</p> <p>}</p> <p>//---------------------------------------------------------<br/> // Loop<br/> //---------------------------------------------------------</p> <p>void loop() {</p> <p>Keyboard.begin(); //begin keyboard<br/> if (digitalRead(2) == 0) // if buton 2 is pushed - Emulates Spotlight Search<br/> {<br/> // For a single keypress use press() and release()<br/> Keyboard.press(KEY_LEFT_GUI); // Presses the Mac Command key<br/> Keyboard.press((char) 32); // Presses the space key<br/> Keyboard.releaseAll();<br/> delay(200); // delay to emulate short press<br/> }<br/> else if (digitalRead(3) == 0) // if button 3 is pressed - Emulates Window Select<br/> {<br/> Keyboard.press(KEY_LEFT_GUI);<br/> Keyboard.press((char) 0x09); // Presses the TAB key<br/> Keyboard.releaseAll();</p> <pre><code>delay(300); </code></pre> <p>}<br/> else if (digitalRead(4) == 0) // if button 4 is pressed - Emulates Save Function<br/> {<br/> Keyboard.press(KEY_LEFT_GUI); // Presses the z key<br/> Keyboard.press('s');<br/> Keyboard.releaseAll();</p> <pre><code>delay(300); </code></pre> <p>}<br/> else if (digitalRead(5) == 0) // if button 5 is pressed - Emulates Redo Function<br/> {<br/> Keyboard.press(KEY_LEFT_GUI);<br/> Keyboard.press(KEY_LEFT_SHIFT);<br/> Keyboard.press('z'); // Presses the z key<br/> Keyboard.releaseAll();</p> <pre><code>delay(300); </code></pre> <p>}<br/> else if (digitalRead(6) == 0) // if button 6 is pressed - Emulates Find Function<br/> {<br/> Keyboard.press(KEY_LEFT_GUI);<br/> Keyboard.press('f'); // Presses the f key<br/> Keyboard.releaseAll();</p> <pre><code>delay(300); </code></pre> <p>}<br/> else if (digitalRead(7) == 0) // if button 7 is pressed - Emulates Undo Function<br/> {<br/> Keyboard.press(KEY_LEFT_GUI); // Presses the shift key<br/> Keyboard.press('z'); // Presses the z key<br/> Keyboard.releaseAll();</p> <pre><code>delay(300); </code></pre> <p>}<br/> Keyboard.end(); //stops keybord<br/> }</p>
With this file you will be able to print Mini HID Keypad with your 3D printer. Click on the button and save the file on your computer to work, edit or customize your design. You can also find more 3D designs for printers on Mini HID Keypad.