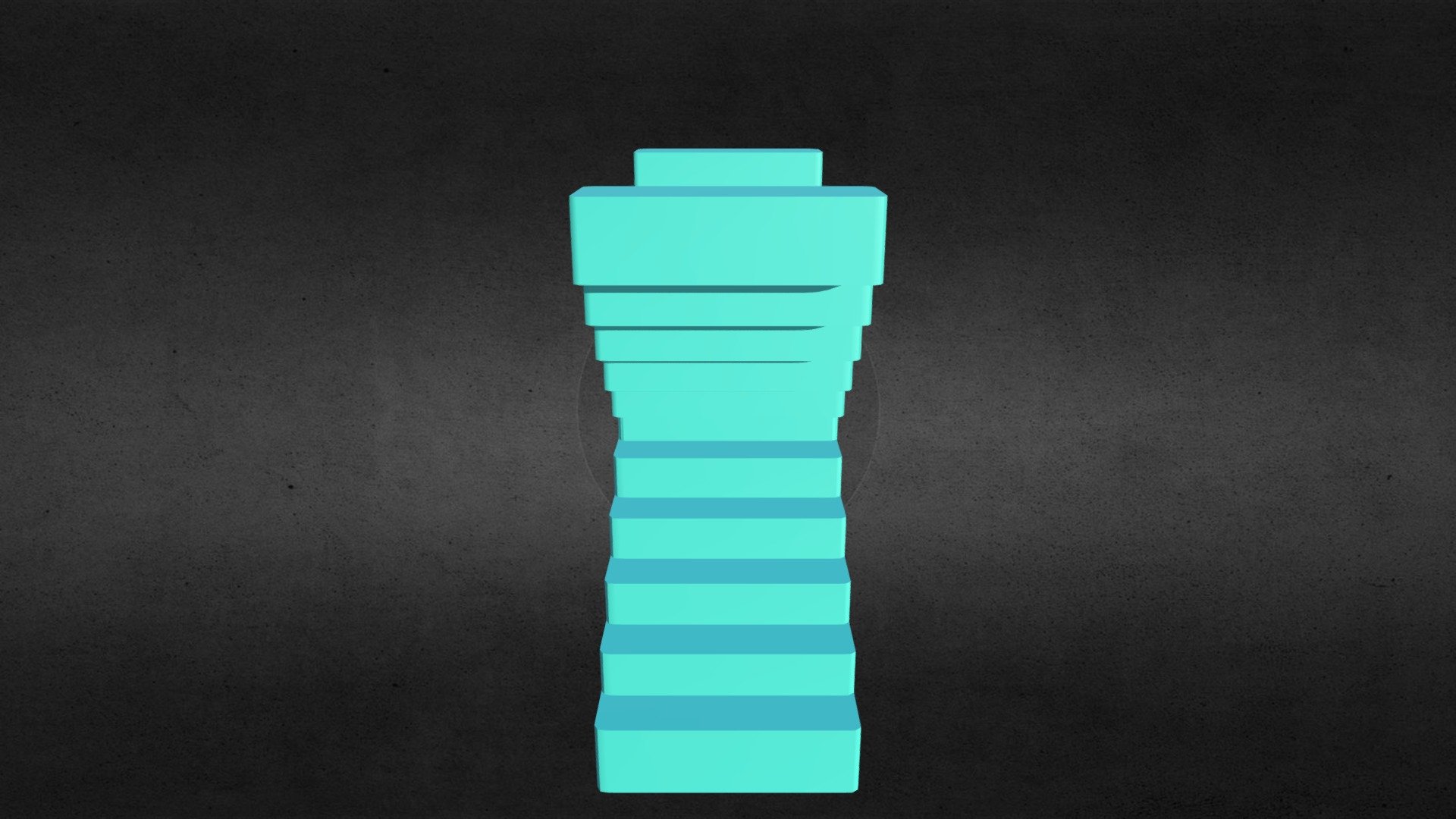
Mario
sketchfab
Creation of Mirror and For Loop in OpenSCAD. OpenSCAD is a powerful tool that allows designers to create complex 3D models using a simple programming language. One of the key features of OpenSCAD is its ability to use loops, which enable users to repeat tasks or actions multiple times. In this context, we will explore how to use the "for" loop and the "mirror" function in OpenSCAD. The "for" Loop: The "for" loop is a fundamental construct in programming that allows us to execute a block of code repeatedly for each item in a list or range. In OpenSCAD, we can use the "for" loop to create arrays of objects or to perform repetitive tasks. Here's an example: ```scad module cylinder(height, radius) { cylinder(h = height, r = radius); } cylinder_heights = [10, 20, 30]; cylinders = []; for (i = [0 : len(cylinder_heights)]) { push(); translate([0, i * 5, 0]); rotate([90, 0, 0]); cylinder(height = cylinder_heights[i], radius = 2); pop(); } ``` In this example, we create an array of cylinder heights and use a "for" loop to iterate over each height. For each iteration, we create a new cylinder with the corresponding height. The Mirror Function: The mirror function in OpenSCAD is used to flip objects or arrays along a specific axis. This can be useful for creating symmetrical models or for reducing the complexity of an object by eliminating redundant parts. Here's an example: ```scad module rectangle(width, depth) { linear_extrude(depth) square([width, width]); } rectangles = [ [10, 20], [30, 40] ]; mirrored_rectangles = []; for (i = [0 : len(rectangles)]) { mirrored_rectangle = mirror(rectangles[i], center = true); push(); translate([-100 + i * 50, 0, 0]); rotate([0, 90, 0]); linear_extrude(10) polygon(mirrored_rectangle); pop(); } ``` In this example, we create an array of rectangles and use a "for" loop to iterate over each rectangle. For each iteration, we mirror the rectangle along the y-axis using the mirror function. Combining the "For" Loop and Mirror Function: By combining the "for" loop and the mirror function in OpenSCAD, we can create complex models with ease. Here's an example: ```scad module cylinder(height, radius) { cylinder(h = height, r = radius); } cylinder_heights = [10, 20, 30]; mirrored_cylinders = []; for (i = [0 : len(cylinder_heights)]) { mirrored_cylinder = mirror(cylinder([cylinder_heights[i], 2]), center = true); push(); translate([-100 + i * 50, 0, 0]); rotate([90, 0, 0]); cylinder(height = cylinder_heights[i], radius = 2); pop(); } ``` In this example, we create an array of cylinder heights and use a "for" loop to iterate over each height. For each iteration, we mirror the corresponding cylinder along the y-axis using the mirror function. Conclusion: The combination of the "for" loop and the mirror function in OpenSCAD provides designers with powerful tools for creating complex 3D models. By mastering these features, users can create intricate designs that were previously impossible to produce.
With this file you will be able to print Mario with your 3D printer. Click on the button and save the file on your computer to work, edit or customize your design. You can also find more 3D designs for printers on Mario.