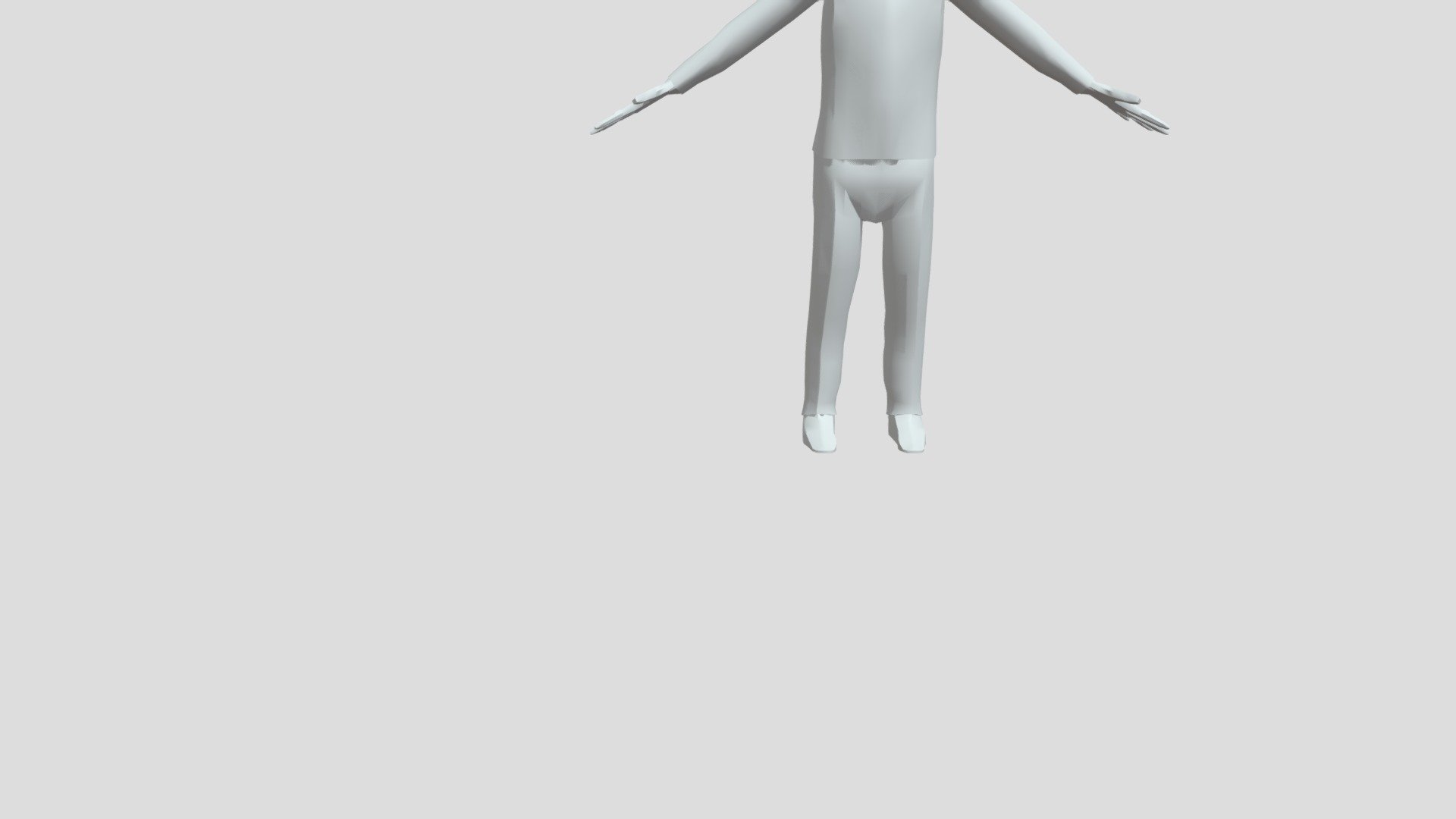
Male
sketchfab
Create a Python Script to Train and Save a Text Classification Model =========================================================== This assignment requires you to train a text classification model using scikit-learn and save it as a pickle file. ### Step 1: Import Libraries Import the necessary libraries, including pandas, numpy, and scikit-learn. ```python import pandas as pd import numpy as np from sklearn.feature_extraction.text import TfidfVectorizer from sklearn.model_selection import train_test_split from sklearn.metrics import accuracy_score from sklearn.linear_model import LogisticRegression ``` ### Step 2: Load the Dataset Load the dataset into a pandas DataFrame. The dataset should contain two columns: 'text' and 'label'. ```python df = pd.read_csv('dataset.csv') ``` ### Step 3: Split the Data Split the data into training and testing sets using `train_test_split`. ```python X_train, X_test, y_train, y_test = train_test_split(df['text'], df['label'], test_size=0.2, random_state=42) ``` ### Step 4: Vectorize the Text Data Vectorize the text data using `TfidfVectorizer`. This will convert the text into a numerical representation that can be used by the machine learning algorithm. ```python vectorizer = TfidfVectorizer() X_train_vectorized = vectorizer.fit_transform(X_train) X_test_vectorized = vectorizer.transform(X_test) ``` ### Step 5: Train the Model Train a logistic regression model on the vectorized training data using `LogisticRegression`. ```python model = LogisticRegression(max_iter=1000) model.fit(X_train_vectorized, y_train) ``` ### Step 6: Evaluate the Model Evaluate the performance of the model on the testing data using `accuracy_score`. ```python y_pred = model.predict(X_test_vectorized) print('Accuracy:', accuracy_score(y_test, y_pred)) ``` ### Step 7: Save the Model Save the trained model to a pickle file using `joblib.dump`. ```python import joblib joblib.dump(model, 'model.pkl') ``` This script will train a text classification model and save it as a pickle file named 'model.pkl'. The model can then be loaded and used for making predictions on new data.
With this file you will be able to print Male with your 3D printer. Click on the button and save the file on your computer to work, edit or customize your design. You can also find more 3D designs for printers on Male.