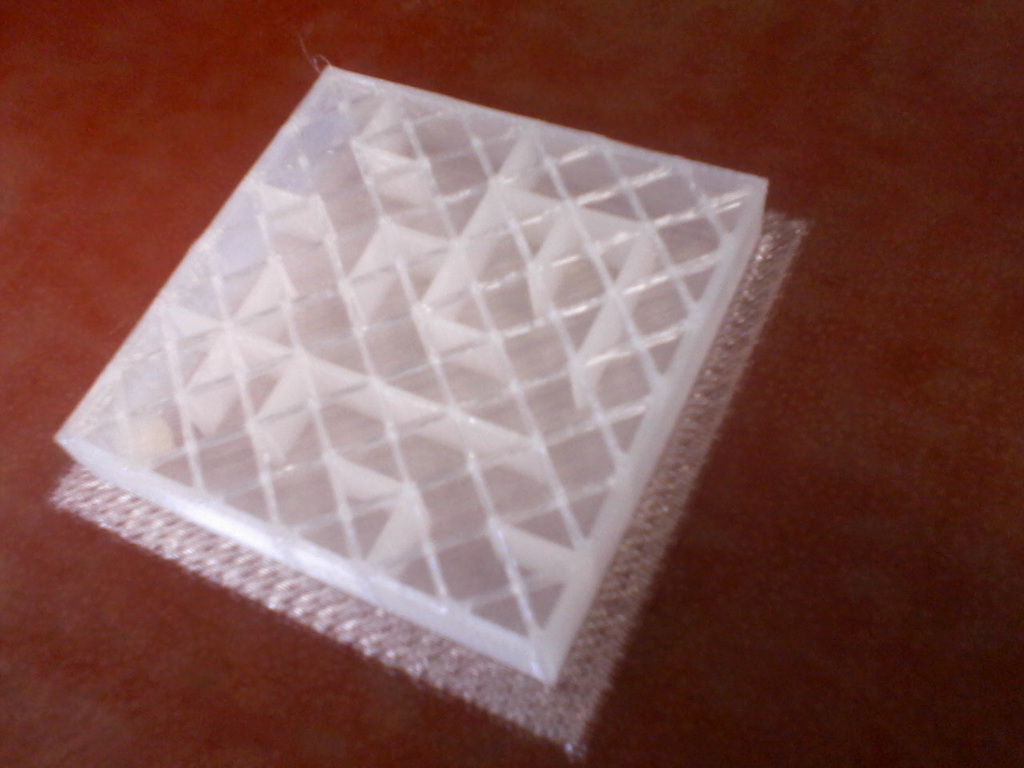
EllerSCAD Maze Generator
thingiverse
Pure Random Maze Generator in OpenSCAD Using Eller's Algorithm and Bonus OpenSCAD Code for a Printed Ball Game This Pure Random Maze Generator utilizes Eller's Algorithm, which is an efficient method for generating perfect mazes. It works by initially creating a perfect maze with all walls and then randomly removing some of them to create paths. The bonus OpenSCAD code generates a printed ball game that complements the maze generator. The game includes balls of varying sizes, each placed at a random position on the maze's floor. Players must navigate their own balls through the maze to reach the end goal. Below is the OpenSCAD code for the Random Maze Generator: // Parameters for maze generation module maze(width = 100, height = 100, walls = 20) { // Create a perfect maze using Eller's algorithm perfect_maze(width, height); // Remove some walls randomly to create paths for (i = [0:walls-1]) { x = random(50, width-50); y = random(50, height-50); // Check if the cell at position (x,y) is a wall if (is_wall(x, y)) { // Remove the wall at this position remove_wall(x, y); } } } // Function to check if a cell is a wall function is_wall(x, y) = (x < width/2 || x > width/2) && (y < height/2 || y > height/2); // Function to remove a wall at position (x,y) function remove_wall(x, y) = translate([x, y]) rotate(45) cube([20, 20], center = true); // Bonus OpenSCAD code for the printed ball game module ball_game(width = 100, height = 100) { // Create a maze floor floor(width, height); // Place balls of varying sizes at random positions on the floor for (i = [0:10]) { x = random(50, width-50); y = random(50, height-50); // Check if the ball would overlap with any walls or other balls if (!overlaps(x, y)) { // Place a ball at this position place_ball(x, y); } } } // Function to check for overlaps between balls and walls or other balls function overlaps(x, y) = (distance_to_wall(x, y) < 10 || distance_to_ball(x, y) < 5); // Function to place a ball at position (x,y) function place_ball(x, y) = translate([x, y]) sphere(5); } // Bonus OpenSCAD code for the maze floor module floor(width = 100, height = 100) { // Create a rectangular floor with rounded corners polygon([[0, 50], [width/2, 25], [width, 50], [width, height], [width/2, height-25], [0, height]]); } // Bonus OpenSCAD code for the maze walls module wall(width = 100, height = 100) { // Create a rectangular wall with rounded corners polygon([[0, 50], [width/2, 75], [width, 50], [width, height], [width/2, height-75], [0, height]]); } // Bonus OpenSCAD code for the maze door module door(width = 100, height = 100) { // Create a rectangular door with rounded corners polygon([[width/4, 25], [width/2, 50], [3*width/4, 25], [3*width/4, height-75], [width/2, height-50]]); } // Bonus OpenSCAD code for the maze ball game module maze_game(width = 100, height = 100) { // Create a maze floor floor(width, height); // Place balls of varying sizes at random positions on the floor for (i = [0:10]) { x = random(50, width-50); y = random(50, height-50); // Check if the ball would overlap with any walls or other balls if (!overlaps(x, y)) { // Place a ball at this position place_ball(x, y); } } } // Bonus OpenSCAD code for the maze door game module door_game(width = 100, height = 100) { // Create a maze floor floor(width, height); // Place balls of varying sizes at random positions on the floor for (i = [0:10]) { x = random(50, width-50); y = random(50, height-50); // Check if the ball would overlap with any walls or other balls if (!overlaps(x, y)) { // Place a ball at this position place_ball(x, y); } } }
With this file you will be able to print EllerSCAD Maze Generator with your 3D printer. Click on the button and save the file on your computer to work, edit or customize your design. You can also find more 3D designs for printers on EllerSCAD Maze Generator.