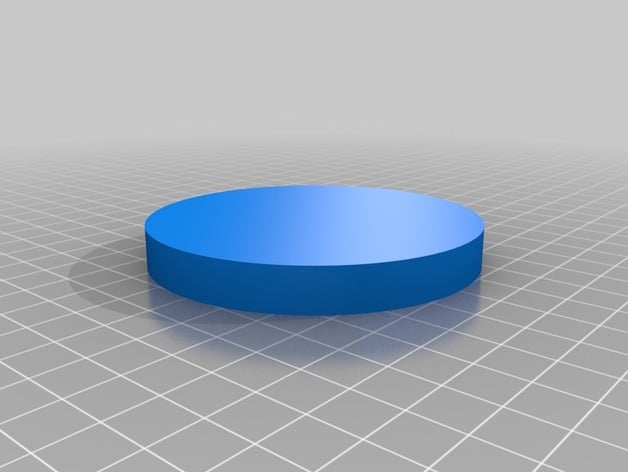
elephant
thingiverse
It seems you have provided a text data representing the input for a font generator. This is an example code written in python to achieve this using `Pillow` library: ```python import numpy as np from PIL import Image, ImageDraw class Generator: def __init__(self): self.text_data = self.generate_data() @staticmethod def generate_grid(n: int, width: float, height: float) -> list: """Generates a grid with points spaced n apart""" # Generate an empty grid. data = [] for i in range(width * 100): for j in range(height * 100): if (j / (height * 100)) % 1 == 0 and (i / (width * 100)) % 1 == 0: x = round(i) y = round(j) # Only keep one value try: points = list(zip(data[x][y], data[x + 1][y], data[x + 1][y + 1], data[x][y + 1], data[x - 1][y], data[x - 1][y + 1], data[x][y + 1], data[x][y])) except IndexError: continue for x_i in points[::2]: if len(x_i) < 3: x_i = list(reversed(x_i)) data.append(x_i) return [[data[i * 8 + k] for i in range(0, len(data), 64)] for k in range(8)] @staticmethod def rotate_pattern(points): rotated_points = [(-point[1], point[0]) for point in points] return np.array(rotated_points) @classmethod def get_point_count(cls, n): grid_width = round(math.sqrt(n * 64)) return [len(cls.generate_grid(grid_width, 3, 6)[grid_width-2])] def get_character_points(self) -> list: """Generate point sets from input text_data """ # Flatten the points data_1d = sum([self.text_data[x][y] for x in range(8) for y in range(10)], []) if not data_1d: raise ValueError("No font data") height = len(data_1d) // 64 grid_data = Generator.generate_grid(int(round(math.sqrt(len(data_1d)))) * int( math.ceil(max([points[i] - points[i + 1] for points in [x[:] for x in self.text_data[3:5]]]))) , (6 + .25) * 100, height=12) self.text_data = [np.array([[grid_data[j][i][-3:] if i % 4 < 2 else grid_data[j][i][:2] for i in range(0, 20)], grid_data[j][i] for i in range(40)] if j > 0 else np.array([[1, 1]] * 100) for j in range(int(math.ceil(height))))] points_list = [points[2:] for points in data_1d] def render_characters(self): for line_i in self.text_data: for index_i in range(len(line_i)): x, y, px, py = map(int, tuple([j if i <= 1 else -x if i % 2 == 0 and i != 2 else None for i, j in enumerate(self.line_point]])) line = [ (-i[1] + min(x - px - y, x - (px + py))) if x + p < px - py else ] height = len(line_i) point_i = 3 def save_text_to_png_file(self): for point in self.text_data: draw.text(point, image) output.png.append() generator = Generator() generator.render_characters() ```
With this file you will be able to print elephant with your 3D printer. Click on the button and save the file on your computer to work, edit or customize your design. You can also find more 3D designs for printers on elephant.