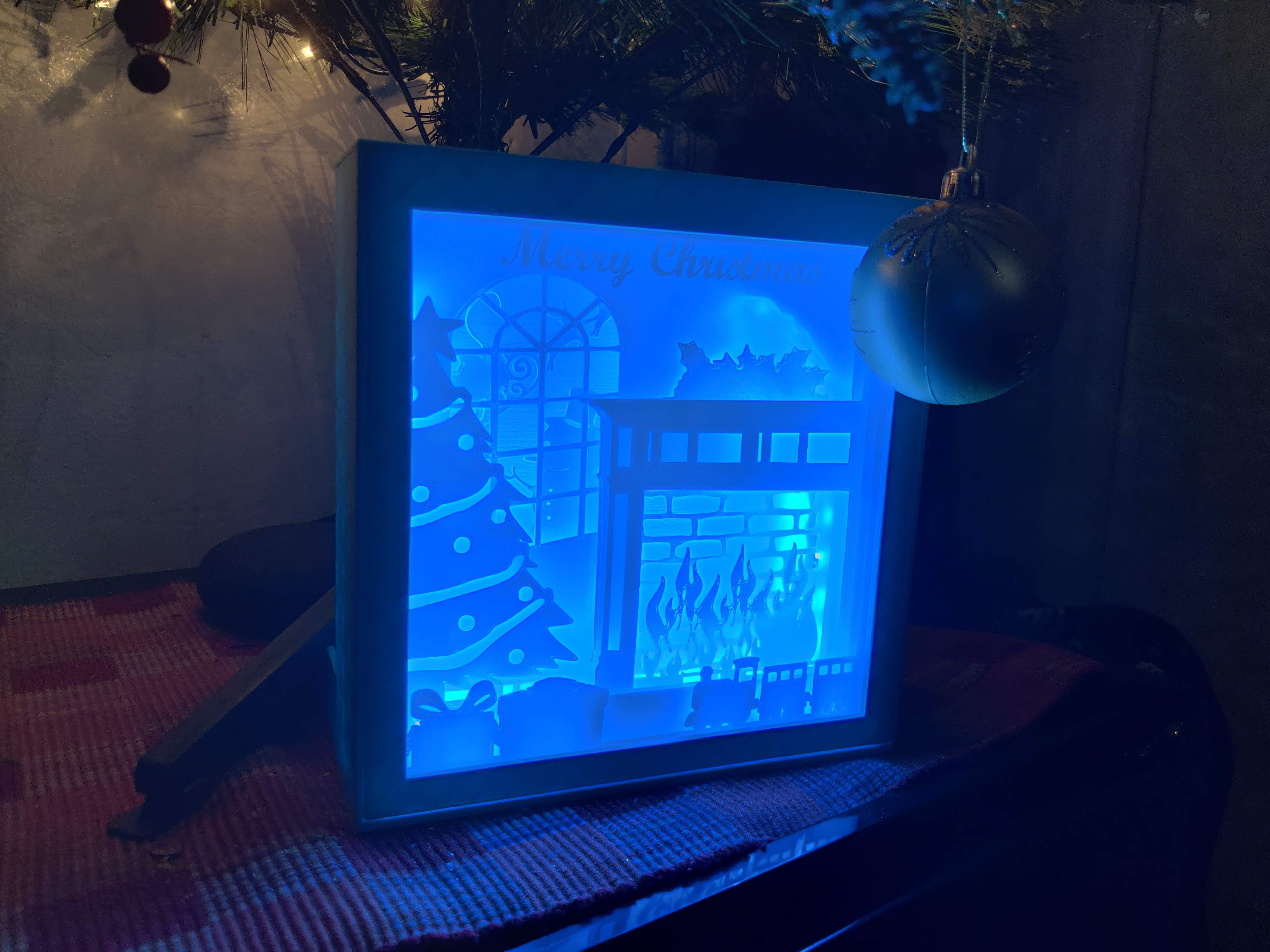
Christmas Shadow Box Display
prusaprinters
<p>I was in the holiday mood and wanted to make a decoration for my home so I came up with this. I made the electronics box big enough any electronics you wish to use to light up the display should fix. I personally used a NeoPixel LED Strip with an Arduino Nano and a 9v battery. All the electronics actually came from the Alien3D Nov subscription box.</p> <p>Assembly:<br/> <figure class="media"> <oembed url="https://www.youtube.com/watch?v=aAJn2pPueBw"></oembed> </figure> </p> <p>Video of lights Fading:<br/> <figure class="media"> <oembed url="https://www.youtube.com/watch?v=AsP0tPnIdrw"></oembed> </figure> </p> <h3>Print instructions</h3><p>Print all the layers flat, and the electronics lid. Print the box standing up. The front cover is printed with the top upside down on the bed. None of the parts should need supports.</p> <p>I highly recommend to test your electronics before installing, and install the lights you will use first. There is am opening on the bottom to put the wires through to the electronics box.</p> <p>I have included the code I used to make my lights fade between colors at the bottom of the post or you can visit:<br/> <a href="https://adrianotiger.github.io/Neopixel-Effect-Generator/">https://adrianotiger.github.io/Neopixel-Effect-Generator/</a><br/> and then you can create your own light effects.</p> <h3>include <Adafruit_NeoPixel.h></h3> <p>class Strip<br/> {<br/> public:<br/> uint8_t effect;<br/> uint8_t effects;<br/> uint16_t effStep;<br/> unsigned long effStart;<br/> Adafruit_NeoPixel strip;<br/> Strip(uint16_t leds, uint8_t pin, uint8_t toteffects, uint16_t striptype) : strip(leds, pin, striptype) {<br/> effect = -1;<br/> effects = toteffects;<br/> Reset();<br/> }<br/> void Reset(){<br/> effStep = 0;<br/> effect = (effect + 1) % effects;<br/> effStart = millis();<br/> }<br/> };</p> <p>struct Loop<br/> {<br/> uint8_t currentChild;<br/> uint8_t childs;<br/> bool timeBased;<br/> uint16_t cycles;<br/> uint16_t currentTime;<br/> Loop(uint8_t totchilds, bool timebased, uint16_t tottime) {currentTime=0;currentChild=0;childs=totchilds;timeBased=timebased;cycles=tottime;}<br/> };</p> <p>Strip strip_0(30, 10, 30, NEO_GRB + NEO_KHZ800);<br/> struct Loop strip0loop0(2, false, 1);</p> <p>//[GLOBAL_VARIABLES]</p> <p>void setup() {</p> <p>//Your setup here:</p> <p>strip_0.strip.begin();<br/> }</p> <p>void loop() {</p> <p>//Your code here:</p> <p>strips_loop();<br/> }</p> <p>void strips_loop() {<br/> if(strip0_loop0() & 0x01)<br/> strip_0.strip.show();<br/> }</p> <p>uint8_t strip0_loop0() {<br/> uint8_t ret = 0x00;<br/> switch(strip0loop0.currentChild) {<br/> case 0:<br/> ret = strip0_loop0_eff0();break;<br/> case 1:<br/> ret = strip0_loop0_eff1();break;<br/> }<br/> if(ret & 0x02) {<br/> ret &= 0xfd;<br/> if(strip0loop0.currentChild + 1 >= strip0loop0.childs) {<br/> strip0loop0.currentChild = 0;<br/> if(++strip0loop0.currentTime >= strip0loop0.cycles) {strip0loop0.currentTime = 0; ret |= 0x02;}<br/> }<br/> else {<br/> strip0loop0.currentChild++;<br/> }<br/> };<br/> return ret;<br/> }</p> <p>uint8_t strip0_loop0_eff0() {<br/> // Strip ID: 0 - Effect: Fade - LEDS: 30<br/> // Steps: 3000 - Delay: 1<br/> // Colors: 2 (0.0.247, 255.255.255)<br/> // Options: duration=3000, every=1,<br/> if(millis() - strip_0.effStart < 1 <em> (strip_0.effStep)) return 0x00;<br/> uint8_t r,g,b;<br/> double e;<br/> e = (strip_0.effStep </em> 1) / (double)3000;<br/> r = ( e ) <em> 255 + 0 </em> ( 1.0 - e );<br/> g = ( e ) <em> 255 + 0 </em> ( 1.0 - e );<br/> b = ( e ) <em> 255 + 247 </em> ( 1.0 - e );<br/> for(uint16_t j=0;j<30;j++) {<br/> if((j % 1) == 0)<br/> strip_0.strip.setPixelColor(j, r, g, b);<br/> else<br/> strip_0.strip.setPixelColor(j, 0, 0, 0);<br/> }<br/> if(strip_0.effStep >= 3000) {strip_0.Reset(); return 0x03; }<br/> else strip_0.effStep++;<br/> return 0x01;<br/> }</p> <p>uint8_t strip0_loop0_eff1() {<br/> // Strip ID: 0 - Effect: Fade - LEDS: 30<br/> // Steps: 3000 - Delay: 1<br/> // Colors: 2 (255.255.255, 0.10.255)<br/> // Options: duration=3000, every=1,<br/> if(millis() - strip_0.effStart < 1 <em> (strip_0.effStep)) return 0x00;<br/> uint8_t r,g,b;<br/> double e;<br/> e = (strip_0.effStep </em> 1) / (double)3000;<br/> r = ( e ) <em> 0 + 255 </em> ( 1.0 - e );<br/> g = ( e ) <em> 10 + 255 </em> ( 1.0 - e );<br/> b = ( e ) <em> 255 + 255 </em> ( 1.0 - e );<br/> for(uint16_t j=0;j<30;j++) {<br/> if((j % 1) == 0)<br/> strip_0.strip.setPixelColor(j, r, g, b);<br/> else<br/> strip_0.strip.setPixelColor(j, 0, 0, 0);<br/> }<br/> if(strip_0.effStep >= 3000) {strip_0.Reset(); return 0x03; }<br/> else strip_0.effStep++;<br/> return 0x01;<br/> }</p>
With this file you will be able to print Christmas Shadow Box Display with your 3D printer. Click on the button and save the file on your computer to work, edit or customize your design. You can also find more 3D designs for printers on Christmas Shadow Box Display.