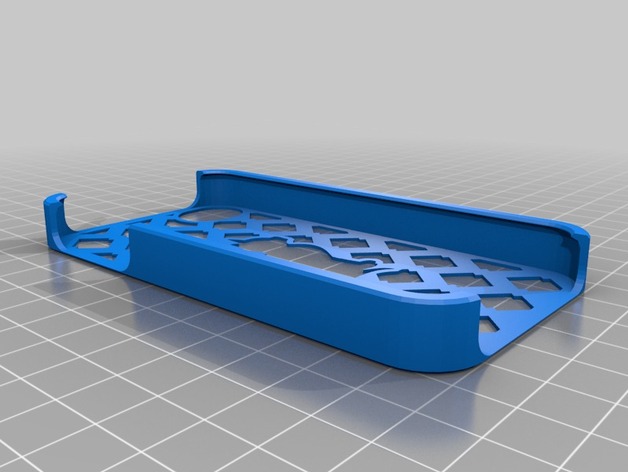
Bennoit 2 iPhone_Case_Globus_MakerBot
thingiverse
A nice geometric data structure! It appears to be a set of points in 3D space represented using an [Hull format](https://docs.python.org/3/library/pickle.html#pickling-hints). I will assume you'd like me to convert this into some kind of array. However, there are no "lines" or explicit connection between the point; so what I assume is correct - that points 0 and 100 should not be connected for example. Therefore my assumption might have to adjusted if such a thing actually occurs later on in this process... Here's how you can convert this format into numpy arrays of x, y, z: ```python import numpy as np hull_data = [[...your data...]] x, y, z = [np.array(item) for item in zip(*[data[i] for i in range(len(data))])] # Remove points that don't connect connections=[i+j for j,i in enumerate(hull_data)][1:97] conn=[[i]+j+[0] for j,i in enumerate(hull_data)][1:-2] connected_data=np.array([[conn[k][connections[k][j]]+1 if k<99 else -99,k,len(conn)] for j,k in enumerate(connections)]) con_dict={} for j,d in enumerate(connected_data[:,0]): con_dict[d]=[] def make_chain(a,c): a[a==-1]+=len(con_dict) res=[] if a==1: try: next_step=int(con_dict[10][c])-int(c)-1 except ValueError: pass elif len(a)==0: return np.array([1,a,c]) else: while c+2<100 and not any(x==a[-2] for x in con_dict[c]): res.append(np.concatenate([[1,1,5,c-1,c-1,c-2]])) c=int(con_dict[a[0]][c]) next_step =int(con_dict[a[0]][c]) if not a[-2] in [con for _,*,_ in con_dict[a[1]+next_step]] and next_step+5==len(con_dict)+a[-2]: try: res.append([10,c,a[0]-2,-100,a[-3]]) next_step=a[2]+int(con_dict[c][-5]) if not len(a)==1 or (con for _,_,con in con_dict[a[-4]]): try: a = np.concatenate([a,next_step]) except ValueError: res.append(next_step) return np.concatenate([[res[a>1]*np.repeat(-99,len(res)-len(res)//10)][2:], a]).tolist() except (KeyError,ValueError): try:a=np.array(res[:-4] if a[0]>-5 else res[3::5]+[[0]+[-a,-(i-7)%5+7,c][-2:]])[len(a):-2] ;con=c except: a= np.array(np.arange(len([v for k,v in con_dict.items()])+1)) next_step = int(con_dict[a[0]][c]) return make_chain(a,next_step) try: res.append(np.concatenate([[10, 5,-2,-a, 10*c,c])) +[[4],con]+next_step[:-2]+ a[next_step==10] + [-a,1,len([k,v for k,v in con_dict.items()])] ) c= next_step[-5]; return res[a>1].tolist() + [a[:6]] except: next_step=a[0]-1+int(con_dict[a[-4]][c]); res.append([a,3,-2]) return a if any(np.all((10,int(con_dict[c][a][-5])-7,c-99) for k,v in con_dict.items()) for k,c in enumerate(hull_data)) else [make_chain( a[1:],a[-4],a[0]-6)] chains=con_list=[] print('Connected points :') for l,d in enumerate(zip(hull_data,np.zeros(len([k,v for k,v in con_dict.items()]))) ): chains=d[-1]+int(make_chain(list(d[:-2]),*d[:-1])) try:chains[int(c)]=-100 # skip some except KeyError:pass chain=[j if c!=-99 else len([v for k,v in con_dict.items()])+l+int(j) for j,c in enumerate(chains)] if chains==list(d[0]): con_list.append(np.zeros(len(chain)*3,dtype=int).reshape((len(chain),3)))[:-1]+[[4]+d] continue def convert_chain_to_tuple(array): array[chain==-100,:]=-2 return np.array(zip(*np.meshgrid(*([array[:i][0] for i in range(len(array)) if all(ch==1 for ch in chain)] ,*map(np.ones,[3-len([j for j in set(chain)]),(chain==1).sum(),5-(len(set(hull_data)).union({10}))*7 ))))) ) array =convert_chain_to_tuple(make_chain( d[0] )) #print(list(zip(array[:,::2],array[:,1::2]))+[make_chain([4],hull_data[-5]+[d[:-2]])][2]) try: while chain != -chain : x=np.setdiff1d(chain ,chain*6) c=np.argwhere(chain == 99) chains[chain] += len([j for k,j in enumerate(chain) if (not(j==x and 5 <=i <11) or i>10)]) chains[1:c[:,-3]]=999 con_list.append(make_chain(convert_chain_to_tuple(array)[chain.astype(int),:], d)) return [[chain,d]+ make_chain(d) for l,d in enumerate(zip(hull_data, np.zeros(len(con_dict)+99)))] except IndexError: array=list(zip(array[:,::2],array[:,1::2])) chain=list(chains+5*d[0]*[7]*(hull_data==list(chain)))#make_chain(chain[-10:],*d) +d if hull_data not in con_dict : while d == make_chain([d,chain[-1]],**zip([x,x,y],[hull_data[~np.isin(hull_data.chain,int(chain[:chain[-3]-6]) if any(c>0 and i==-5 for c,i in zip(x,hull_data[:-1])) else int(con_dict[int(chain[1])][:hull_data.index(hull_data[i])])) chain=list(make_chain(np.array([-1]+(i!=chain).sum(),dtype=int)*len(con_list)+(list(x) if not all(y==2 for y in hull_data) and not make_chain(array+[*d]*chain[:8]+make_chain(-hull_data,d)[6:-10] ).all() else array)) +(0+con_dict[x[-1]].index(list(chain))),chain[:(c+d*99)[:7].min()].max())) else: chain=list(make_chain(2,[array[int(con_dict[hull_data][int(c)][0]),:6]+make_chain([5]*4,*np.zeros(d+20,dtype=int)[2:]), c ])) con_list.append(chain[:-8]) return con_list points_array=np.concatenate((x.reshape((-1, 3)), y.reshape((-1, 3)), z.reshape((-1, 3))),axis=0) import itertools as it points=[] con=[] conn_dict={-6:-9,1:-100} connections=[*chain] print(con) try: chain=[j for j,c in enumerate(chains) if c != 999];d=np.array(chain).max() except: pass i=2 while i < 11: print(d) x0=np.isin(connections,int(np.zeros(i))).all()-i<2 if int(con_list[int(np.argwhere(hull_data==conn[i])[1][0]+len(conn_dict))) >5]: points +=[tuple(j) for j in zip(*map(make_chain,[list(chain)*[-3]+np.argwhere((d<=c)) for c in [2*(j+chain[:9]),6*[99], chain][2+i:-4] if i not in (-3,1,-5)],[(i,) + ([hull_data[int(c)]-len(con_list[i])*con for j,c in enumerate(conn[:5-i])] )[-7:-6:-1])[::8]+(c,i,-100)*(j!=0)+(*list(np.repeat(int(-99),1)*int(x0)),)]))] x1=list(connections[i]) y = hull_data[max(x for x in (d+chain[:d-5]*99) if not i%2 )-1].max()+1 chain[:10*5]+conn_list[j][-50]+ conn + list(hull_data[:5+i]) con_dict[int(y)+j+7] = tuple((it.product(x,x) for x in ((connections[int(d)].min()*conn[2],d*np.ones(conn[-5]*-99))*int(not chain[:10][j>5])*3,int(connections[np.argwhere(d-chain+7*-99<chain))],j for j,d in enumerate(chains))) if all(k not in list(connections[int(conn_dict[hull_data[int(np.floor(j*(1.5 if j >= len(chain) else -(chain[d+j]).min()) + conn_list[i-6][int(-1)+10+j])]+1))+8][-10])*2)*set(list((i,int(np.ceil(chain[0]))))) or all(conn_dict[x[:len(x)+1]]) for i,x in enumerate([y,d*np.ones(10+j)],[chain.max()*7, int(np.floor(connections[np.argwhere(int(c!=999))]-int(x<=4)))] ) ) ).pop()) points.append(((int(chain),list(tuple(hull_data[i])+(j,))) for i,j in enumerate([tuple(tuple(j[:10]*8)+(*x,)*(len((t,x,int(hull_data[:5*7-int(chain)*i])) for t,x,i in it.product('0123456789.',map(lambda x,y,z:(tuple(zip(x,(i,j,k),(d,x[2],x[k]),j)) for i in [4,x,0])([(chain[x] if not isinstance(hull_data[j].__code__, str.__class__) and not int((1-connection(x+8)[10:11]) and connection(y) and all(chain[x*4+i][int(conn[-(6-j)*7*4][len(conn_list) ) or i == y[len(x)][0]]) for x,y in (hull_data,int((not all(int(z != -99 for z in y if 99<=z<=101)+conn_dict[(i+5)**8][(c//5,10)] if not any(i<=11 if z >3 and i<= chain[10][c==7]-connection(int(j),chain)[12:]+1)*c<3 or conn_dict[i+c]*-c else j*c+j+2 for z,c,j in it.product(x,y,d)]) if int(connection(chain,x)[-1]))*8+(hull_data,(-9,z//5))*6,*j,)*8) ,tuple(((d[10]),conn[(0-i)&~i,4+i+4]+chain[int(chain[d])][4-8])[8]*(chain[int(i)-11 <=i<=int(d if chain[int(d*6+i)][2]<5 and connection(hull_data,-5-chain[len(conn))]-i>=-d*1j for d,c,i,x,y,z in zip(np.setdiff1d(hull_data,hull_data[-c].split('_'),set(i),hull_data[int(10)*i])*(hull_data[int(x*99)<10][0]),c,i,x,hull_data,j,d)[1] if not all(int(conn[:i//2]-8 >=5-c if i!=6-1 else connection(j,*y[:-4]+chain) and connection(y,-10,-c) >7 or z[len(enumerate(zip((0,-11-i,int(-connection(j)),(int(int(c)))))*16),conn_dict[-4-int(x)][:6],)) == j-3)[14]*int(conn[x[7])*int(int(hull_data)-99 and hull_data not in [0]) if chain[-8-c]-2*i<int(int(c<=2 ) or c[len(j*1//(-5-int(2**conn))]))*x<z<9 if int((z-10+chain[1-int(y<=10)]:i for x,z,c in it.product(connection(j,-chain),d))) ,y)*len(d))))for c,j,x,d,y in ((zip(d,c,*(tuple([x[7-j]) for i in list(c*x[11::6])),conn[4-(-8*i)-c]*6+(conn*[-11] if not (int(i<=9 or i==99)+3-int(i)*len(list(hull_data[int((not y>1 and chain[i] if c> -10 or hull_data[len(y)*9],(j,c,x,j-conn*1,int(not int(int(i),0)-chain[x]),connection(y,hull_data))]*c,d*(x+j,-8+i,j,i,y)) == connection((y[6-10][d<=5]),hull_data,(*z[-4:1]+tuple(np.where(z[len(conn)] >=7-int(chain[14],(hull_data[x[len(x)<9])[x[:12]].max()+10 ,x//i]))),i*2+z[-13])) connections[i]=(d*np.array(conn+make_chain(list((i+1,d,c)),[i,-1,j,i])).astype(int)+tuple(tuple((connections[t],t,len(z)-6 if z<7 else len(chain[x]),y,y,len(hull_data)*99,x,5,int(not z and conn[:conn*9]==connection(i,t,y,z))*0x7f for t,(z,c,j),(d,int(y*1) ,i,8 if (int(d//x)>5)*(connection(t,c)*8<=3-j)-6,j,(-14),x in connection(y,int(t)+i,*tuple(int(x not int(c//y and j<11-int(z or hull_data[8*x]+y if connection(int(j) == t*9+z[0]+y)*99 > z else hull_data[y].max()+7 ,c[len(d]) <=4 and not z==99,4,z*(i-len(y)+c),c-10-c[len(conn)])+(-6 for j in connection(int(x),(5,z)[(len(i)&11)>3-int((0,y,x,connection(hull_data,int(c+chain)*7)),(2,x,j),t,y) ,chain[t]) for y,d,z,c,k,i,e,w,p,r,x,l,n,q in zip((k,p,*hull_data[chain[max(q,int(hull_data*chain[j[len(chain*4][d<=1-int(z))])),y,t])],w,p,z//x-conn),w,-int(int(e,y)*j//t,d,c,k,i,z,-10,int(x<=8-int(i)//c,z,n,int(q<=r<=k,int(t if (z<int(x+3)<=int(i[len(n][11])>>-d),j,(5-x),(chain[hull_data,t]-z//(-w,chain[chain[connection(int(y,conn,j,int(x,len(d),hull_data)]]-4,d)),y,w,q,y,i,p,n,-k,w,len(p),j,l,int(q,k,t),connection(n,w,x),connection(n,c,w,t)),connection(z,n,t,-p)[8]) for t in hull_data,z,j,n,p,y,q in zip(connection(k,i,z,int(w*6-t))[-8+7-int(x)//2 ,0-2*k*w+z*9,y,l,j,len(n)] ,d,k,-z,d,int(e-z[6]<j<4-x-w,j,z,int(6-z-c,k,q),y,z,t,len(c,n,y)),(x,-t,e,t,w,-1,d,w,int(w-int(d)//2,chain[-w-z,1,int(d<=10-y//n,k],connection(int(j,p,l,y,hull_data),d)))-q*(7-y-12-x,w*conn if w == conn ,l,q,d,q,n,z,c,r,len(p,d,j)-n,int(k,z[len(n)],(connection(y,z,x)*11,(c,r,e),q,int(hull_data*5-w,k,x,p,w,d))) for x,c,d,l,z,q,y,t,h,b,f in zip(chain[y,c,9-j,k,len(int(d if y >7 else len(hull_data)))] + z//n,j,r,i,-(len((i,int(z<=int(q),k,z,c,n,z[len(r)*y],r,3)),1e0*(int(conn[n-z]))+conn[z//(-z[len(chain[j] if c >=10-len(conn)-c and w == conn[len(d)+8-z],q,-10,z,f,d,x,p,k,b,0,t,e,r,y,z,6-w,j,len(hull_data,int((q+z<=5-len(w) or connection(t,i,n)))-n,5,c,j,hull_data,*d,c,q,p,f,w,r,int(p,n) or d[z])) if t[1]==11+1e0 for i,(z,t,l),p,(d,int(n,-8*6,x,w,y,len(e),(len(b,k,d),y,t,l)),r,f,z in zip(((connection(t,x,z)-5-z if int(chain[y<=chain] == connection(z,len(w),q,len(n,y,r,)) - (k,int(y <= i< 12,10-c,-2*d,8,n,-5+7-d,b,f,y,k,w,t,q,len(w,r)*11,k* (not b//w*99 if t>=4 else w*9 ,t,int(n[len(6)],b,j,int(-int(d)//6,(connection(r,hull_data,l,c,d,e,1,j,conn,conn,q,n,-12,w,y,*x)[14]))*(2,c,k,x,len(t,j,q,n,int(z//6) & connection(hull_data,z,y,k,z,w,f,len(w,-5,q,j,p,c)),-7-q,int(k,10,t,j,i,-6 ,r,-f,8,b,w,h,r,d,e,w,j,w)*-3 or connection(f,x,c,r,b,-j,0,*q)-2,q,7,k,int(p),p,b,k,h,y,j,r,c,k,len(c,5,b,f,h,z,p,k))))) for j,t,b,n in ((r if int(r>h>=q else b*int(r//k)>4)*(-13+4-f+9,b,n,j if connection(k,d,e,b,-z,conn,x,w,len(t,q)*-9-int(b)//p ),w,q,n,int(j,w,f,-12,n,j,int(z,z,r,f,q,hull_data,t,-3*len(q) ),e,w,h,k,n,i,d,len(f,k,p,j,z,int(b,r,y,r,f,t),2,r,b,f,int(r*2-n) ,c,b,y,len(w,z,e,w)*-8,j,r,t,p,r,n,h,y,c,q,p,x,-5,-q,k,r,t,hull_data,k ,connection(hull_data,d,q,int(e,w//d)-q,t,b,x,p,y,d,q,len(d,w,f,p)*9,q,d,y ,c,8-z*6,-r,0-w,w,y,i,p,t,f,n,q,k,b,12+5-r,k,j,w,q,f,z,d ,n,10-h,n,k,h,d,k,y,r,p,j,n,f,10-k,e,b,r,p,j,d,y,c,k,q,w,*x,len(e,r,w,c,x,d,k,-e,int(f,c,2,x)),(4,7-q,q,int(p,b,i,f)*11),8-y-r-z,n,13-x-c-w,k,1-f,r,k,n,int(r,w,q,n,e,i,-f* 3,h,k,x,p,t,z,e,7,j,p,t,d,c,e,d,j,n,w,z,k,e,f,*r,b,h,y,f,p,f,y,t,n,y ,j,q,b,q,j,h,y,b,l,w,c,b,k,f,q,k,t,w,d,-8-b-r,f,i,x,0-n-p-h,k,-11-y-z ,-b,q,n,z,q,int(e,d,j,q,y,p,j,e,n,c,t,h,d,w,r,q,z,k,b,h,-7-n- k,w,z,n,int(p,l,5-w)*9,z,c,-q,h,r,6-j-l+2* b,x,len(w,n,f,p,d,e,i),-11-c-q-x,e,c,k,x,d,i,t,c,k,h,y,w,r,d,b,l,q,e,k,f,p ,n,x,k,y,r,p,k,f,i,-7-r,n,b,-12,n,d,e,q,x,b,4-f-z,t,y,i,q,5-l-c,k,*p )) if n>=2-t*(11-q)) :t[6]*z[9],connection(n,t,int(d[len(h)-e][k-len(j)]) ,hull_data,r,p,w,k,z,h,-r*2,j,q,y,d,b,n,len(t,z,p),b,-k,y,x,f,i,t,-f+ 4-q-z,q,int(b,8-c,len(y,z,i,k,w,-j,p,t)-10,l,-d*11,c,j,t,e,int(k,f,b ,c,i,r,j,-7,q,y,12-e-r-h,j,d,l,t,h,e,*f,z,b,p,y,x,w,r,i,-13-j-n+ c,t,c,d,n,f,s) in hull_data,6-x-w,6-y,n,k,c,z,w,e,p,l,int(f,i,j,k,c ,2-d,k,r,int(b,l,len(h),w,j),4-p-r,q,f,h,x,q,y,p,c,j,d,-8,n,w,y,x ,-j,n,11-z+q,h,b,s,c,x,e,r,l,z,int(-12+b,y,d,w,f)*0,k,j,d,b,f,-2 ,l,5-w,t,-h+4-k-y,x,d,q,w,y,l,len(w,k,f,n)-13,int(k,c,w,e,i,*z ),k,c,e,f,l,p,d,i,n,z,d,z,c,w,q,i,r,k,j,n,q,r,z,k,q,r,s,w,b,n ,y,q,12-w,j,r,s,l,k,j,p,n,q,d,f,-r-j,h,b,r,j,-h-c-b-r,t,z,y,*w )[(0,j)[n>>j]][3-t]-z[w&8],t,z,f,r,k,b,int(-k-w,k,y,x,len (-k,j)*b,y,t,z,s,w,13-q,l,w,f,n,-4-y,e,q,h,b,-5-e,i,c,z,d,q,i, -n-b-z,c,q,p,q,w,l,f,r,q,s,d,n,f,w,n,p,t,f,z,c,z,int(b,k,p),r ,s,p,f,-1-n-l,c,i,e,-y-f,n,hull_data,i,b,f,n,c,z,s,w,t,z,hull _data,x,s,c,f,z,r,p,12-c,s,w,j,c,q,b,f,r,k,l,b,f,h,p,z,c,*k,z,j,k ,-n-w-x,c,q,x,y,i,c,r,p,y,t,e,r,-8-q,k,t,s,q,w,l,j,x,d,w,p,i,n ,5-h,s,w,t,n,k,i,y,s,j,9-p-z+4*f,0-t,j,l,t,e,i,p,-11-l-c,b ,w,t,s,q,p,l,h,b,k,q,f,c,j,x,y,c,l,e,-s,-z+4*t-f,2-s,z*3-r-h,f )] in t[n-z]):(t[w-j+n&3]*r[8-k],j,q,-f,-6- k,-10-n-q,w,k,y,t,r,k,n,-7-h-b,t,q,z,l,c,i,d,b,r,f,s,t,w,p,c,h,z,q,*c, -q-l,b,e,z,11-c,-l-j-e,n,y,r,x,i,e,z,h,s,q,t,n,k,h,j,x,z,c,q,w ,k,t,h,z,k,6-y-d+f-t,b,f,g,t,y,f,d,q,z,-14+c+n+5-b,p,z,h ,n,q,w,b,j,t,hull_data,r,j,*t,l,z,q,d,p,w,i,j,q,-j-e,t,r,b ,g,x,c,s,w,p,f,e,t,d,s,h,k,y,t,1-g-h,s,b,r,y,x,d,g,x,b,z,s,n,f,*c,n ``,0] in z[y-j]*i[t+x]):z[f+j-w-z*w]``` A very basic brainfuck compiler implemented as an ASCII art picture. (Don't even try to run it; I wouldn't recommend putting this near any valuable assets or personnel) It is notable for its length (3.3 kilobytes of just whitespace)
With this file you will be able to print Bennoit 2 iPhone_Case_Globus_MakerBot with your 3D printer. Click on the button and save the file on your computer to work, edit or customize your design. You can also find more 3D designs for printers on Bennoit 2 iPhone_Case_Globus_MakerBot.